반응형
import calendar
calendar.prmonth(2007,7)
July 2007
Mo Tu We Th Fr Sa Su
1
2 3 4 5 6 7 8
9 10 11 12 13 14 15
16 17 18 19 20 21 22
23 24 25 26 27 28 29
30 31
calendar.prmonth(2007,7)
July 2007
Mo Tu We Th Fr Sa Su
1
2 3 4 5 6 7 8
9 10 11 12 13 14 15
16 17 18 19 20 21 22
23 24 25 26 27 28 29
30 31
import turtle import *
reset()
forward(50)
left(90)
reset()
forward(50)
left(90)
>>> import keyword
>>> dir(keyword)
['__all__', '__builtins__', '__doc__', '__file__', '__name__', 'iskeyword', 'kwlist', 'main']
>>> keyword.kwlist
['and', 'as', 'assert', 'break', 'class', 'continue', 'def', 'del', 'elif', 'else', 'except', 'exec', 'finally', 'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'not', 'or', 'pass', 'print', 'raise', 'return', 'try', 'while', 'with', 'yield']
>>>
>>> dir(keyword)
['__all__', '__builtins__', '__doc__', '__file__', '__name__', 'iskeyword', 'kwlist', 'main']
>>> keyword.kwlist
['and', 'as', 'assert', 'break', 'class', 'continue', 'def', 'del', 'elif', 'else', 'except', 'exec', 'finally', 'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'not', 'or', 'pass', 'print', 'raise', 'return', 'try', 'while', 'with', 'yield']
>>>
>>> eval('4*5*6-4+7')
123
>>>
123
>>>
>>> name = raw_input('이름? : ')
이름? : 전호철
>>> name
'\xc0\xfc\xc8\xa3\xc3\xb6'
>>>
>>> num = raw_input("number : ")
number : 789
>>> number
Traceback (most recent call last):
File "<input>", line 1, in <module>
NameError: name 'number' is not defined
>>> num
'789'
>>> int(num)
789
>>> float(num)
789.0
>>>
이름? : 전호철
>>> name
'\xc0\xfc\xc8\xa3\xc3\xb6'
>>>
>>> num = raw_input("number : ")
number : 789
>>> number
Traceback (most recent call last):
File "<input>", line 1, in <module>
NameError: name 'number' is not defined
>>> num
'789'
>>> int(num)
789
>>> float(num)
789.0
>>>
>>> for x in range(5):
... print x
...
0
1
2
3
4
>>> for x in range(5):
... print x, #, 붙이면 가로로 출력
...
0 1 2 3 4
>>>
... print x
...
0
1
2
3
4
>>> for x in range(5):
... print x, #, 붙이면 가로로 출력
...
0 1 2 3 4
>>>
>>> L = [1, 2, 3, 'ham', 'na']
>>> len(L)
5
>>> 'na' in L
True
>>> L.append('j')
>>> L
[1, 2, 3, 'ham', 'na', 'j']
>>> dir(L)
['__add__', '__class__', '__contains__', '__delattr__', '__delitem__', '__delslice__', '__doc__', '__eq__', '__ge__', '__getattribute__', '__getitem__', '__getslice__', '__gt__', '__hash__', '__iadd__', '__imul__', '__init__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__', '__setattr__', '__setitem__', '__setslice__', '__str__', 'append', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort']
>>> L[1:3]
[2, 3]
>>>
>>> len(L)
5
>>> 'na' in L
True
>>> L.append('j')
>>> L
[1, 2, 3, 'ham', 'na', 'j']
>>> dir(L)
['__add__', '__class__', '__contains__', '__delattr__', '__delitem__', '__delslice__', '__doc__', '__eq__', '__ge__', '__getattribute__', '__getitem__', '__getslice__', '__gt__', '__hash__', '__iadd__', '__imul__', '__init__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__', '__setattr__', '__setitem__', '__setslice__', '__str__', 'append', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort']
>>> L[1:3]
[2, 3]
>>>
>>> D={'one':1, 'two':2}
>>> D['one']
1
>>> D['two']
2
>>> dir(D)
['__class__', '__cmp__', '__contains__', '__delattr__', '__delitem__', '__doc__', '__eq__', '__ge__', '__getattribute__', '__getitem__', '__gt__', '__hash__', '__init__', '__iter__', '__le__', '__len__', '__lt__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__setitem__', '__str__', 'clear', 'copy', 'fromkeys', 'get', 'has_key', 'items', 'iteritems', 'iterkeys', 'itervalues', 'keys', 'pop', 'popitem', 'setdefault', 'update', 'values']
>>> D['one'] = 'hana'
>>> D
{'two': 2, 'one': 'hana'}
>>> D[2:1]
Traceback (most recent call last):
File "<input>", line 1, in <module>
TypeError: unhashable type
>>> D['Three']=3
>>> D
{'Three': 3, 'two': 2, 'one': 'hana'}
>>> del D['two']
>>> D
{'Three': 3, 'one': 'hana'}
>>>
>>> D['one']
1
>>> D['two']
2
>>> dir(D)
['__class__', '__cmp__', '__contains__', '__delattr__', '__delitem__', '__doc__', '__eq__', '__ge__', '__getattribute__', '__getitem__', '__gt__', '__hash__', '__init__', '__iter__', '__le__', '__len__', '__lt__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__setitem__', '__str__', 'clear', 'copy', 'fromkeys', 'get', 'has_key', 'items', 'iteritems', 'iterkeys', 'itervalues', 'keys', 'pop', 'popitem', 'setdefault', 'update', 'values']
>>> D['one'] = 'hana'
>>> D
{'two': 2, 'one': 'hana'}
>>> D[2:1]
Traceback (most recent call last):
File "<input>", line 1, in <module>
TypeError: unhashable type
>>> D['Three']=3
>>> D
{'Three': 3, 'two': 2, 'one': 'hana'}
>>> del D['two']
>>> D
{'Three': 3, 'one': 'hana'}
>>>
튜플 : 값을 변경할수 없고, 메소드가 없다.
>>> T=(1,3,'y')
>>> T
(1, 3, 'y')
>>> dir(T)
['__add__', '__class__', '__contains__', '__delattr__', '__doc__', '__eq__', '__ge__', '__getattribute__', '__getitem__', '__getnewargs__', '__getslice__', '__gt__', '__hash__', '__init__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__rmul__', '__setattr__', '__str__']
>>> T[2:2]
()
>>> T[2:]
('y',)
>>> T=(1,3,'y')
>>> T
(1, 3, 'y')
>>> dir(T)
['__add__', '__class__', '__contains__', '__delattr__', '__doc__', '__eq__', '__ge__', '__getattribute__', '__getitem__', '__getnewargs__', '__getslice__', '__gt__', '__hash__', '__init__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__rmul__', '__setattr__', '__str__']
>>> T[2:2]
()
>>> T[2:]
('y',)
시퀀스형 자료형은 슬라이싱, len 등이 가능하다
>>> a=5
>>> exec 'a=a+5'
>>> a
10
>>>
>>> exec 'a=a+5'
>>> a
10
>>>
>>> type(D)
<type 'dict'>
>>> type(L)
<type 'list'>
>>> type(T)
<type 'tuple'>
>>>
>>> type(L)==type([])
True
<type 'dict'>
>>> type(L)
<type 'list'>
>>> type(T)
<type 'tuple'>
>>>
>>> type(L)==type([])
True
>>> kkk='a'
>>> if kkk == 'b':
... print 'b'
... elif kkk=='a':
File "<input>", line 3
elif kkk=='a':
^
SyntaxError: invalid syntax
>>> if kkk == 'b':
... print 'b'
... elif kkk=='a':
... print'a'
... else:
... print 'what!!'
...
a
>>>
>>> if kkk == 'b':
... print 'b'
... elif kkk=='a':
File "<input>", line 3
elif kkk=='a':
^
SyntaxError: invalid syntax
>>> if kkk == 'b':
... print 'b'
... elif kkk=='a':
... print'a'
... else:
... print 'what!!'
...
a
>>>
>>> for x in range(10):
... if x>3: break
... print x
...
0
1
2
3
>>> for x in range(10):
... if x<8: continue
... print x
...
8
9
>>>
... if x>3: break
... print x
...
0
1
2
3
>>> for x in range(10):
... if x<8: continue
... print x
...
8
9
>>>
>>> range(10)
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
>>> range(1,10)
[1, 2, 3, 4, 5, 6, 7, 8, 9]
>>> range(1,11)
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
>>> range(1,50,5)
[1, 6, 11, 16, 21, 26, 31, 36, 41, 46]
>>>
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
>>> range(1,10)
[1, 2, 3, 4, 5, 6, 7, 8, 9]
>>> range(1,11)
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
>>> range(1,50,5)
[1, 6, 11, 16, 21, 26, 31, 36, 41, 46]
>>>
for 조건식 :
문1
else :
문2
위와 같이 되었을 경우 for가 다 돌고 나면 else에 있는 것을 실행하고 나온다.
하지만 for 문에 break이 걸려서 나올 경우에는 else에 있는 것을 생략하고 나간다.
문1
else :
문2
위와 같이 되었을 경우 for가 다 돌고 나면 else에 있는 것을 실행하고 나온다.
하지만 for 문에 break이 걸려서 나올 경우에는 else에 있는 것을 생략하고 나간다.
유니코드 개행문자 \012
>>> a=8
>>> 8
8
>>> a>>1
4
>>> a>>1
4
>>> a<<1
16
>>> a<<1
16
>>> a<<2
32
>>> b=2
>>> b&3
2
>>> b|3
3
>>> ~b
-3
>>> b
2
>>>
>>> 8
8
>>> a>>1
4
>>> a>>1
4
>>> a<<1
16
>>> a<<1
16
>>> a<<2
32
>>> b=2
>>> b&3
2
>>> b|3
3
>>> ~b
-3
>>> b
2
>>>
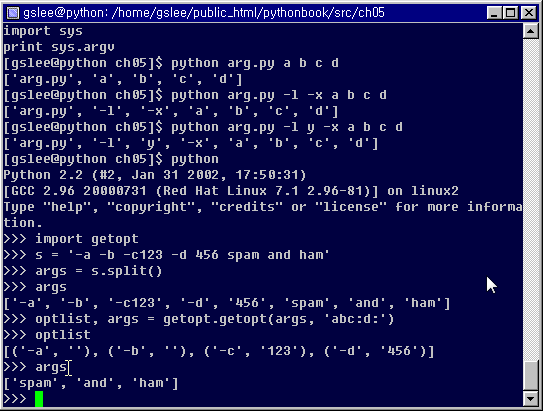
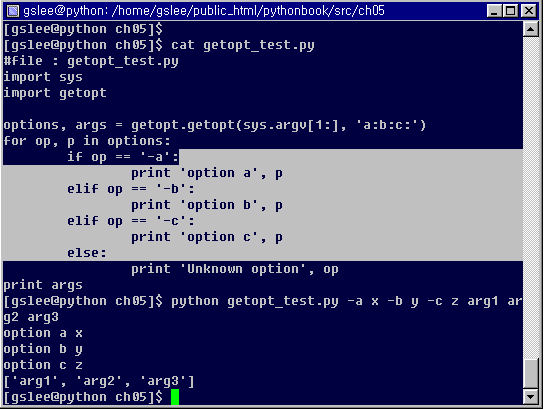
>>> def add(a,b):
... return a+b
...
>>> def sub(a,b):
... return a-b
...
>>> action = {0:add, 1:sub}
>>> action[0]
<function add at 0x01C9AF70>
>>> add
<function add at 0x01C9AF70>
>>> action
{0: <function add at 0x01C9AF70>, 1: <function sub at 0x01C9AFB0>}
>>> action[0](3,4)
7
>>> action[1](3,4)
-1
>>>
>>> action.keys()
[0, 1]
>>> action.values()
[<function add at 0x01C9AF70>, <function sub at 0x01C9AFB0>]
>>> action.items()
[(0, <function add at 0x01C9AF70>), (1, <function sub at 0x01C9AFB0>)]
>>>
>>> action.has_key(1)
True
>>> action.has_key(2)
False
... return a+b
...
>>> def sub(a,b):
... return a-b
...
>>> action = {0:add, 1:sub}
>>> action[0]
<function add at 0x01C9AF70>
>>> add
<function add at 0x01C9AF70>
>>> action
{0: <function add at 0x01C9AF70>, 1: <function sub at 0x01C9AFB0>}
>>> action[0](3,4)
7
>>> action[1](3,4)
-1
>>>
>>> action.keys()
[0, 1]
>>> action.values()
[<function add at 0x01C9AF70>, <function sub at 0x01C9AFB0>]
>>> action.items()
[(0, <function add at 0x01C9AF70>), (1, <function sub at 0x01C9AFB0>)]
>>>
>>> action.has_key(1)
True
>>> action.has_key(2)
False
>>> from Numeric import *
>>> a=array(((1,2,3),(4,5,6),(7,8,9)))
>>> b=a+1
>>> a
array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
>>> b
array([[ 2, 3, 4],
[ 5, 6, 7],
[ 8, 9, 10]])
>>> dir(Numeric)
Traceback (most recent call last):
File "<stdin>", line 1, in ?
NameError: name 'Numeric' is not defined
>>> Numeric.__doc__
Traceback (most recent call last):
File "<stdin>", line 1, in ?
NameError: name 'Numeric' is not defined
>>> matrixmultiply(a,b)
array([[ 36, 42, 48],
[ 81, 96, 111],
[126, 150, 174]])
>>> from LinearAlgebra import *
>>> inverse(a)
array([[ 3.15221191e+15, -6.30442381e+15, 3.15221191e+15],
[ -6.30442381e+15, 1.26088476e+16, -6.30442381e+15],
[ 3.15221191e+15, -6.30442381e+15, 3.15221191e+15]])
>>> a=array(((1,2,3),(4,5,6),(7,8,9)))
>>> b=a+1
>>> a
array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
>>> b
array([[ 2, 3, 4],
[ 5, 6, 7],
[ 8, 9, 10]])
>>> dir(Numeric)
Traceback (most recent call last):
File "<stdin>", line 1, in ?
NameError: name 'Numeric' is not defined
>>> Numeric.__doc__
Traceback (most recent call last):
File "<stdin>", line 1, in ?
NameError: name 'Numeric' is not defined
>>> matrixmultiply(a,b)
array([[ 36, 42, 48],
[ 81, 96, 111],
[126, 150, 174]])
>>> from LinearAlgebra import *
>>> inverse(a)
array([[ 3.15221191e+15, -6.30442381e+15, 3.15221191e+15],
[ -6.30442381e+15, 1.26088476e+16, -6.30442381e+15],
[ 3.15221191e+15, -6.30442381e+15, 3.15221191e+15]])
>>> def f(width, height, **kw):
... print width, height
... print kw
...
>>> f(width=10, height=11, t=5, y=7)
10 11
{'y': 7, 't': 5}
>>>
... print width, height
... print kw
...
>>> f(width=10, height=11, t=5, y=7)
10 11
{'y': 7, 't': 5}
>>>
>>> def printf(format, *args):
... print format % args
...
>>> printf("I am %s", 'sam')
I am sam
>>> printf("I am %s", 6)
I am 6
... print format % args
...
>>> printf("I am %s", 'sam')
I am sam
>>> printf("I am %s", 6)
I am 6
>>> f= lambda a, b : a+b
>>> f(6,8)
14
>>>
>>> f(6,8)
14
>>>
>>> L=filter(lambda x: 0==(x%5) or 0==(x%7) ,range(100))
>>> L
[0, 5, 7, 10, 14, 15, 20, 21, 25, 28, 30, 35, 40, 42, 45, 49, 50, 55, 56, 60, 63, 65, 70, 75, 77, 80, 84, 85, 90, 91, 95, 98]
>>>
>>> L
[0, 5, 7, 10, 14, 15, 20, 21, 25, 28, 30, 35, 40, 42, 45, 49, 50, 55, 56, 60, 63, 65, 70, 75, 77, 80, 84, 85, 90, 91, 95, 98]
>>>
반응형
'Python' 카테고리의 다른 글
에릭 하게만 시리즈 1 - 수치처리 파이썬의 기초 (0) | 2007.08.02 |
---|---|
파이썬으로 레이싱걸 갤러리 긁어오기 (0) | 2007.08.01 |
py2exe (0) | 2007.07.09 |